Starting point for this post is source code for last one, available on bitbucket.
I want to replace dropdown list with jQuery autocomplete, like in this example. There are two reasons for this:
- When there is very large number of records to choose from, then standard dropdown list is not very user friendly
- When user is entering name of item, besides autocompleting from known items, we can implement addition of new item if item user needs to enter is not present in codebook. This will be done in next post.
For start, I will copy example from jQuery demo, and add textbox in which I will implement this functionality.

I have added this code to Index.chtml, which is label for manufacturer, hidden field with id for chosen value from dropdown, and textbox for autocomplete. I have added three attributes for this textbox:
– autocomplete-for is target (hidden field) into which I want to place chosen value
– from-url is address where I will get items for autocomplete
– parent is field for cascading functionality (this will be optional)
Client side code for autocomplete is based on jsonp example from jQuery ui demo:
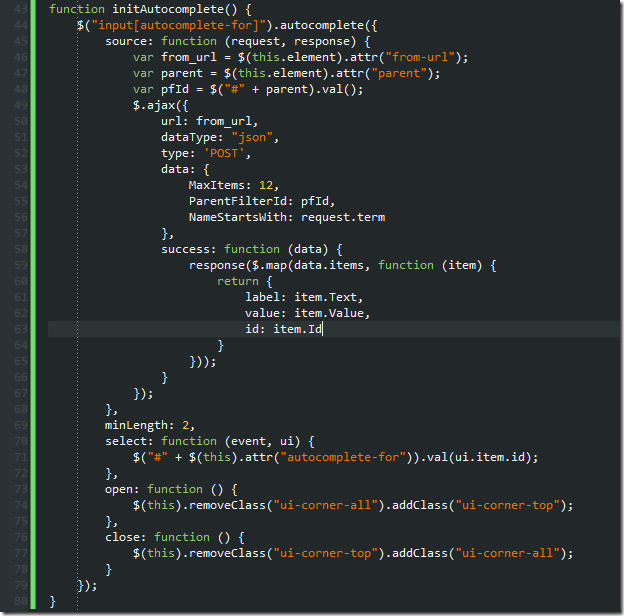
I have added this init function to document ready event. What is happening here is simple: for each input element that has “autocomplete-for” attribute, jQuery autocomplete is added, with custom function as data provider. This function is using ajax to request data for dropdown from from-url specified in view. Parameters are MaxItems (amount of items to show in autocomplete), ParentFilterId (selected value of parent field – for cascading support) and NameStartsWith, which is text entered into dropdown which is autocompleted.
Success function maps “items” collection in response to label, value and id. label and value are automatically used by autocomplete plugin to show label for item in dropdown and to set textbox value when item is selected, and I added id to set hidden field in select event.
To make this work, there is one thing left: controller action which will return items. As you can see in first screenshot, I decided to use Manufacturer controller and action named Autocomplete.
To make parameter passing easier, I have created model for data passed in request:
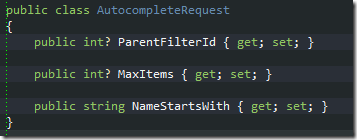
I will receive this object as parameter in my action.
This is how implementation of autocomplete action looks:

This is fairly simple – query is filtered by name for NameStartsWith parameter, and I used ToLower method of string, to compare case insensitive, as StartsWith with string comparison options parameter is not supported in linq to entities.
If request contains parent filter id or max items number, then these are taken into account, and response is formed as collection of items with Text, Value and Id properties, which are used in success function of ajax request.
Time to see how it works:
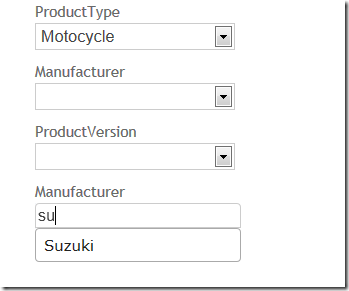
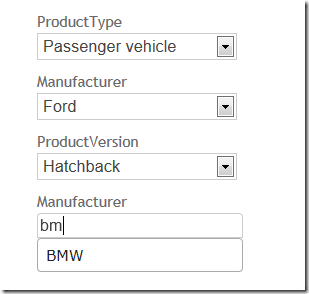
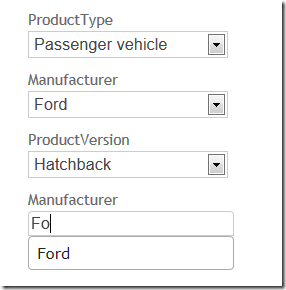
As this is working nicely, time for little makeup, as I want this more reusable, and not having to remember html attributes that need to be used. So, that smells like html helper:
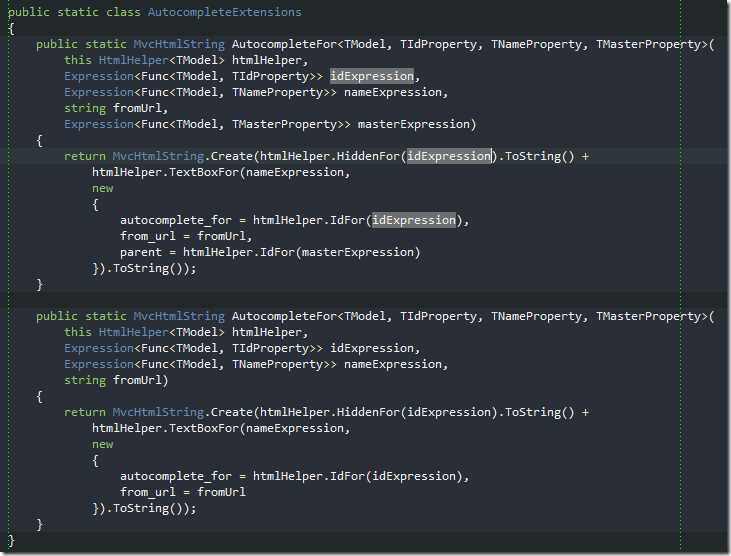
Actually, it is two helpers – one for cascading case, and one for “normal”. It is the same code as in cshtml view, but creating hidden and text field in one method, and when I add comments here (removed to make screenshot smaller), I will have nice intellisense to use this function whenever I need, only having to copy and adjust controller action, which also can be made pretty generic.
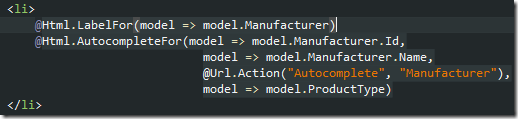
This is how autocomplete is used now. I’m ready to call this a day, and source code from this post is in this changeset on bitbucket.